
getchar()、putchar():
#include < cstdio >
#include < cstdlib >
int main() {
printf("請輸入一個字元:");
char c = getchar();
putchar(c);
putchar('\n');
system("PAUSE");
return 0;
}
getchar()取得使用者輸入的字元,putchar()輸出一個字元。
gets()、puts():
gets()會取得使用者的輸入字串,不包括按下Enter的換行字元碼,puts()用來輸出字串,並直接進行換行。

#include < cstdio >
#include < cstdlib >
int main(void) {
char str[20];
puts("請輸入字串:");
gets(str);
puts("輸入的字串為:");
puts(str);
system("PAUSE");
return 0;
}
格式控制器、格式旗標(cout)
cout有預設的輸出格式,也可以自行指定格式,像endl就是格式控制器的一種,它會輸出new line字元至串流中,格式控制器只會影響目前正在處理的串流,串流處理結束後即回復成預設的格式,格式控制器也可以指定參數,如果要使用具有參數的格式控制器,必須含入iomanip這個標頭檔案,下面為簡單的使用。
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
cout << oct << 30 << endl; //8進位顯示
cout << hex << 30 << endl; //16進位顯示
cout << setw(2) << 5 << endl; //寬度為2
system("PAUSE");
return 0;
}
格式控制器可以改變目前的串流格式,如果想要讓所有的串流都維持特定格式,可以使用格式化旗標,使用setf()與unsetf()來設定與取消格式化旗標,下面為簡單的示範。
#include <iostream>
using namespace std;
int main() {
cout.unsetf(ios::dec);
cout.setf(ios::hex);
cout << 120 << endl;
cout << 50 << endl;
cout.setf(ios::dec);
cout.setf(ios::scientific);
cout << 50.25 << endl;
system("PAUSE");
return 0;
}
Basic types of Variables
Each variable while declaration must be given a datatype, on which the memory assigned to the variable depends. Following are the basic types of variables,
bool | For variable to store boolean values( True or False ) |
char | For variables to store character types. |
int | for variable with integral values |
float and double are also types for variables with large and floating point values |
Declaration and Initialization
Variable must be declared before they are used. Usually it is preferred to declare them at the starting of the program, but in C++ they can be declared in the middle of program too, but must be done before using them.
For example:
int i; // declared but not initialised
char c;
int i, j, k; // Multiple declaration
Initialization means assigning value to an already declared variable,
int i; // declaration
i = 10; // initialization
Initialization and declaration can be done in one single step also,
int i=10; //initialization and declaration in same step
int i=10, j=11;
If a variable is declared and not initialized by default it will hold a garbage value. Also, if a variable is once declared and if try to declare it again, we will get a compile time error.
int i,j;
i=10;
j=20;
int j=i+j; //compile time error, cannot redeclare a variable in same scope

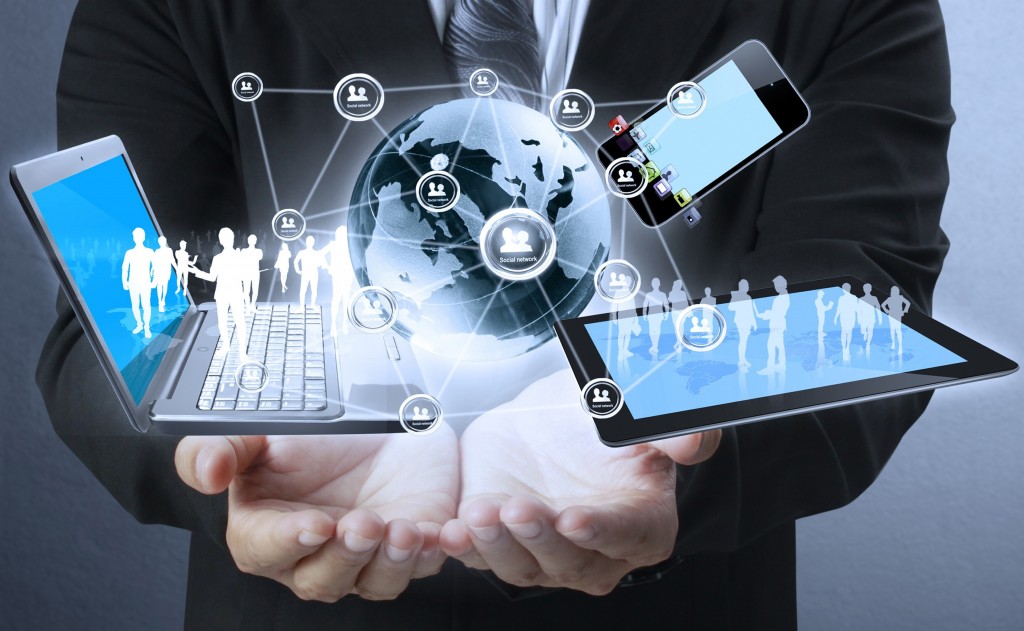
Leave a Reply