To add trailing zeros to a string up to a certain length in Python, convert the number to a string and use the ljust(width, '0')
method. Call this method on the string, specifying the total desired width
and the padding character '0'
. This will append zeros to the right of the string until the specified width
is achieved.
Challenge: Given an integer number. How to convert it to a string by adding trailing zeros so that the string has a fixed number of positions.
Example: For integer 42, you want to fill it up with trailing zeros to the following string with 5 characters: '42000'
.
In all methods, we assume that the integer has less than 5 characters.
Method 1: string.ljust()
In Python, you can use the str.ljust()
method to pad zeros (or any other character) to the right of a string. The ljust()
method returns the string left-justified in a field of a given width, padded with a specified character (default is space).
Below is an example of how to use ljust()
to add trailing zeros to a number:
# Integer value to be converted i = 42 # Convert the integer to a string s = str(i) # Use ljust to add trailing zeros, specifying the total width and the padding character ('0') s_padded = s.ljust(5, '0') print(s_padded) # Output: '42000'
In this example:
str(i)
converts the integeri
to a string.s.ljust(5, '0')
pads the strings
with zeros to the right to make the total width 5 characters.
This is the most Pythonic way to accomplish this challenge.
Method 2: Format String
The second method uses the format string feature in Python 3+ called f-strings or replacement fields.
Info: In Python, f-strings allow for the embedding of expressions within strings by prefixing a string with the letter
"f"
or "F"
and enclosing expressions within curly braces {}
. The expressions within the curly braces in the f-string are evaluated, and their values are inserted into the resulting string. This allows for a concise and readable way to include variable values or complex expressions within string literals.
The following f-string converts an integer i
to a string while adding trailing zeros to a given integer:
# Integer value to be converted i = 42 # Convert the integer to a string and then use format to add trailing zeros s1 = f'{str(i):<5}' s1 = s1.replace(" ", "0") # replace spaces with zeros print(s1) # 42000
The code f'{str(i):<5}'
first converts the integer i
to a string. The :<5
format specifier aligns the string to the left and pads with spaces to make the total width 5. Then we replace the padded spaces with zeros using the string.replace()
function.
Method 3: List Comprehension
Many Python coders don’t quite get the f-strings and the ljust()
method shown in Methods 1 and 2. If you don’t have time to learn them, you can also use a more standard way based on string concatenation and list comprehension.
# Method 3: List Comprehension s3 = str(42) n = len(s3) s3 = s3 + '0' * (5-len(s3)) print(s3) # 42000
You first convert the integer to a basic string. Then, you concatenate the integer’s string representation to the string of 0
s, filled up to n=5
characters. The asterisk operator creates a string of 5-len(s3)
zeros here.
Programmer Humor
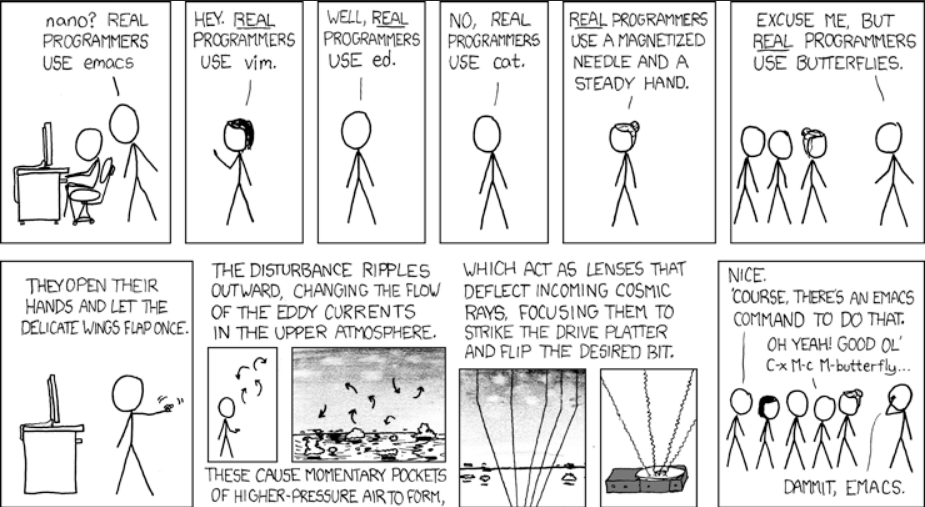
Recommended: Python Int to String with Leading Zeros
The post Python Int to String with Trailing Zeros appeared first on Be on the Right Side of Change.

facebook 查詢:
24 hours enquiry facebook channel :
https://www.facebook.com/itteacheritfreelance/?ref=aymt_homepage_panel
Leave a Reply